Code sample for this is available here! https://github.com/lancelarsen/AutoVersionInFooter
So in days long ago… for ASP.NET websites we were able to set a parameter in our AssemblyInfo.cs to [assembly: AssemblyVersion(“1.0.*”)] — this allowed for auto incrementation of the version number when the project was built.
Why? Well this allowed us to do things like include the version number on the site so we could easily see what version was deployed to what server. Pretty nice feature. 🙂 Something like this…
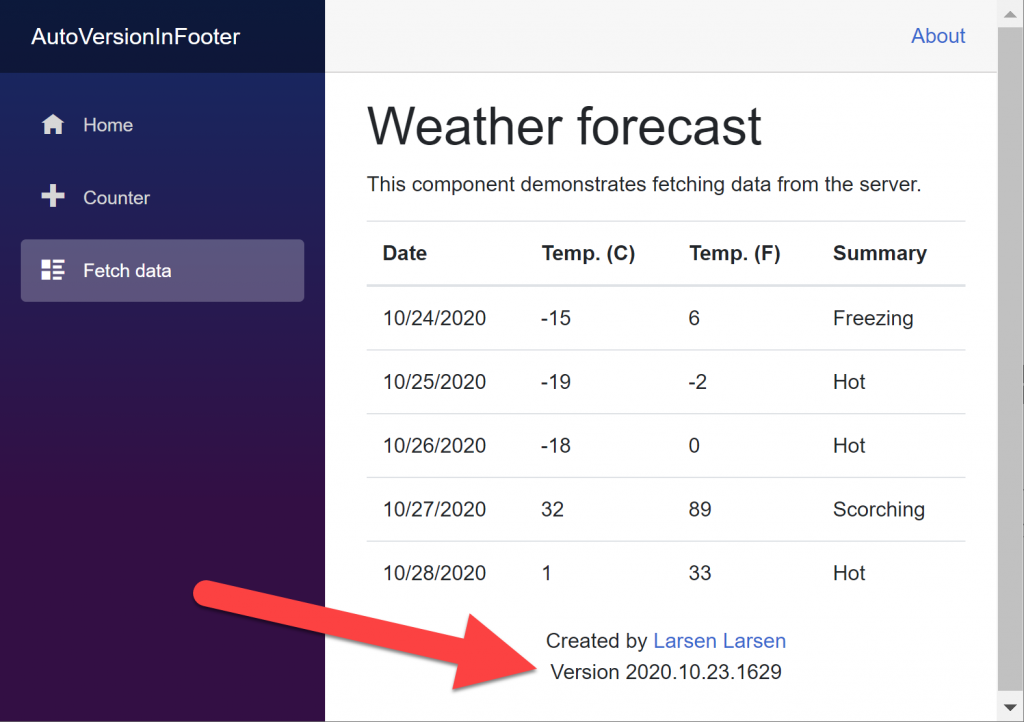
This however is not possible with ASP.NET Core apps — or more importantly the best tool that has become available to .NET web devs — namely Blazor!
Now that we know what’s not available — what do we do to make this possible? Easy… powershell to rescue!
- In your Blazor WebAssembly app — click on YourProjectName.Client
- Verify that you see the <Version>1.0.0</Version> tag — if not, add it.
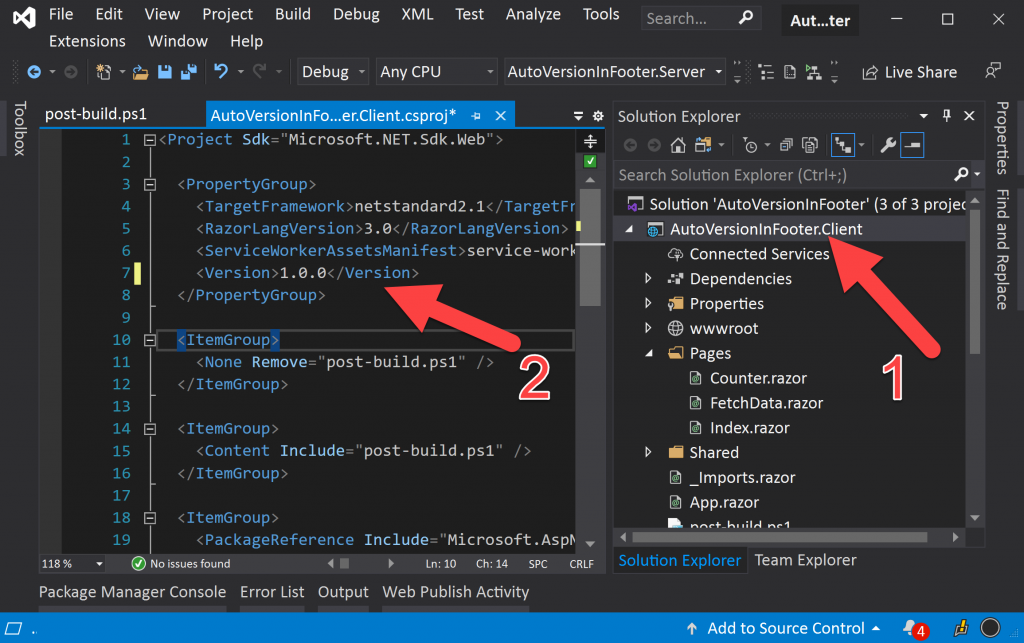
3. Add a Razor Component in your Shared folder — call it SiteFooter.razor
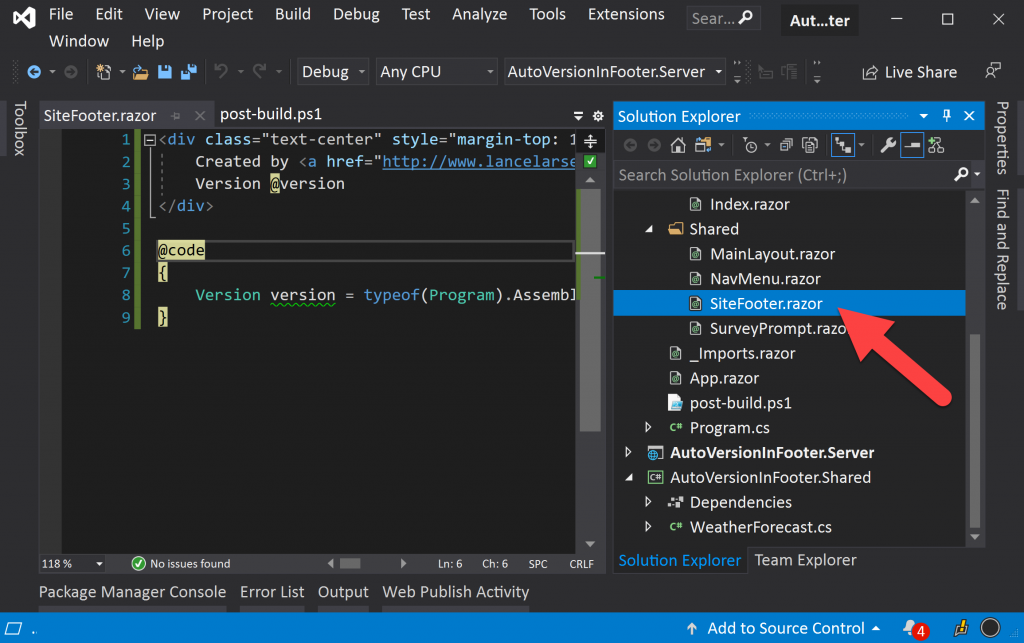
4. Add the following code to the SiteFooter.razor
<div class="text-center" style="margin-top: 10px; margin-bottom: 30px;">
Created by <a href="http://www.lancelarsen.com" target="_blank">Larsen Larsen</a><br/>
Version @version
</div>
@code
{
Version version = typeof(Program).Assembly.GetName().Version;
}
What does it do? Simple — the @version gets the “1.0.0” from the <Version>1.0.0</Version> tag that is in your *.client.config file that we added in step 2. That’s nice — but we want more automation!
5. For display purposes – let’s add the <SiteFooter /> component tag to the bottom of each of the default template pages.
6. Click on FetchData.razor
7. Add <SiteFooter /> component tag. Do the same for Counter.razor and Index.razor
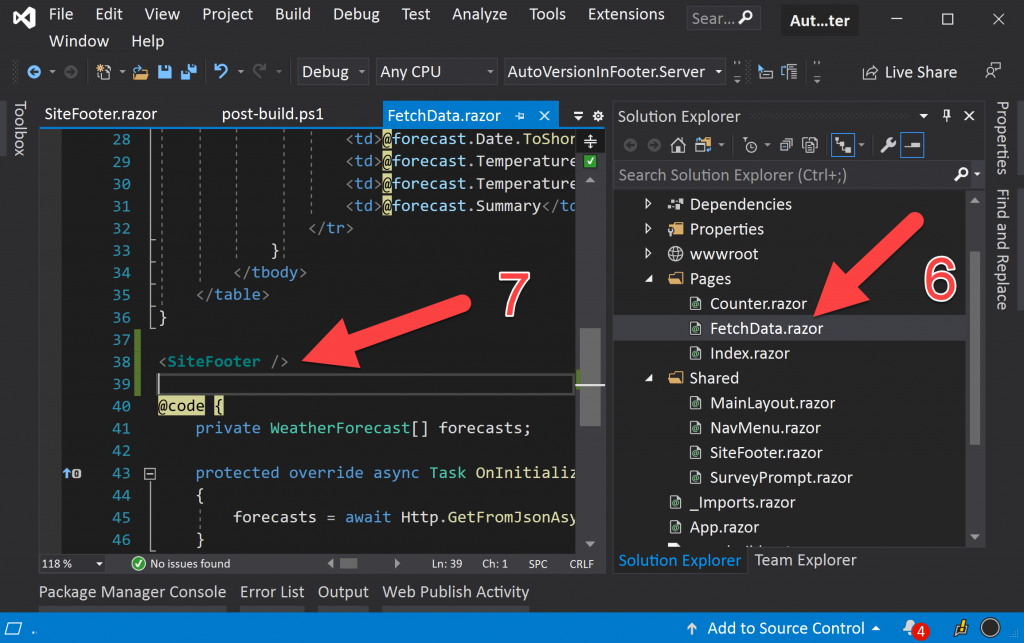
Almost done!
8. Add a new file into the root of your YourProject.Client directory named post-build.ps1
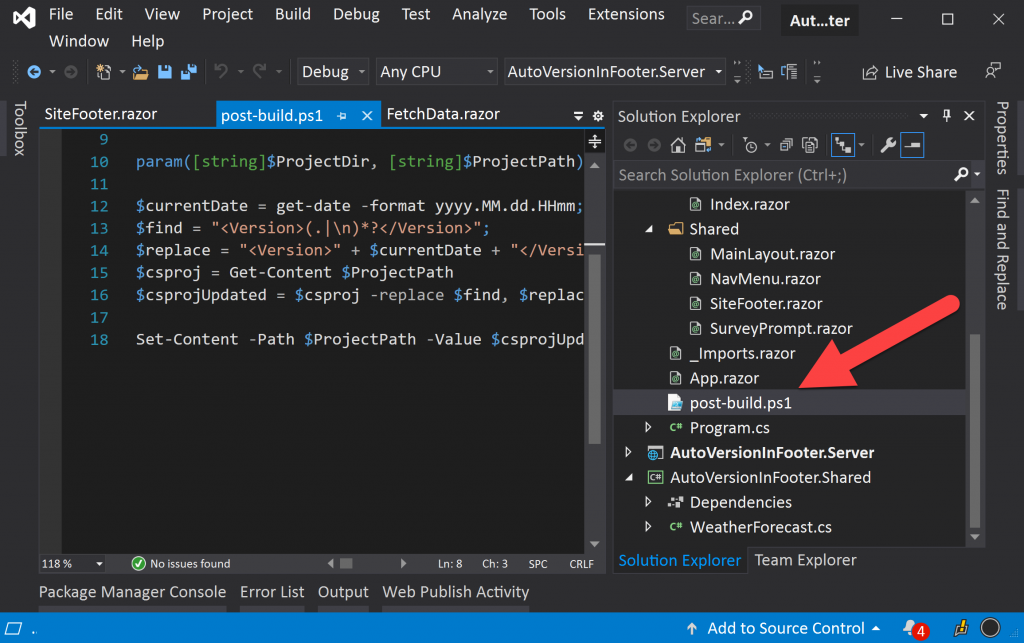
9. Add the following powershell commands into your post-build.ps1 file
param([string]$ProjectDir, [string]$ProjectPath);
$currentDate = get-date -format yyyy.MM.dd.HHmm;
$find = "<Version>(.|\n)*?</Version>";
$replace = "<Version>" + $currentDate + "</Version>";
$csproj = Get-Content $ProjectPath
$csprojUpdated = $csproj -replace $find, $replace
Set-Content -Path $ProjectPath -Value $csprojUpdated
What is this? This is powershell scripting — without going into too much detail, we are essentially getting the current date — formatting it in year.month.day.hour/min format. We then search our *.config file for the <Version>1.0.0</Version> tag and replace it with the current date format. Then finish that up by updating the *.config file. Like magic!
10. Finish by clicking again on your YourProjectName.Client (as we did in step 1) and adding the following…
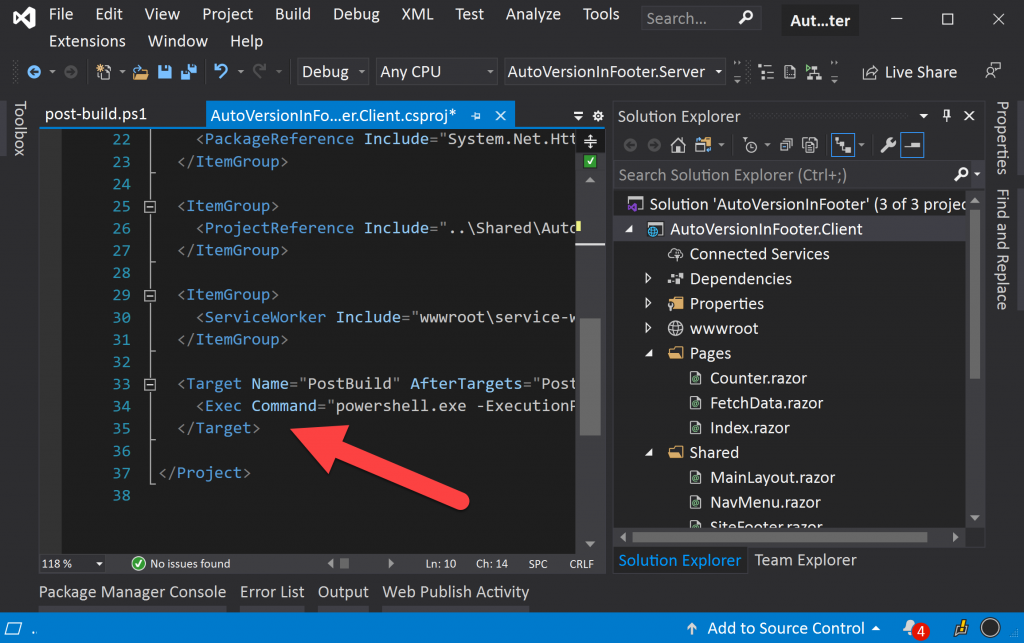
<Target Name="PostBuild" AfterTargets="PostBuildEvent">
<Exec Command="powershell.exe -ExecutionPolicy Unrestricted -NoProfile -NonInteractive -File $(ProjectDir)\post-build.ps1 -ProjectDir $(ProjectDir) -ProjectPath $(ProjectPath)" />
</Target>
What does this do? Executes the powershell command and passes parameters to the post-build.ps1 file – including the path to our *.csproj file that we want to update.
11. Done! Now if you build your project — you should see the <Version>1.0.0</Version> change to something like <Version>2020.10.23.1717</Version>. Every time you build you should see a new value!
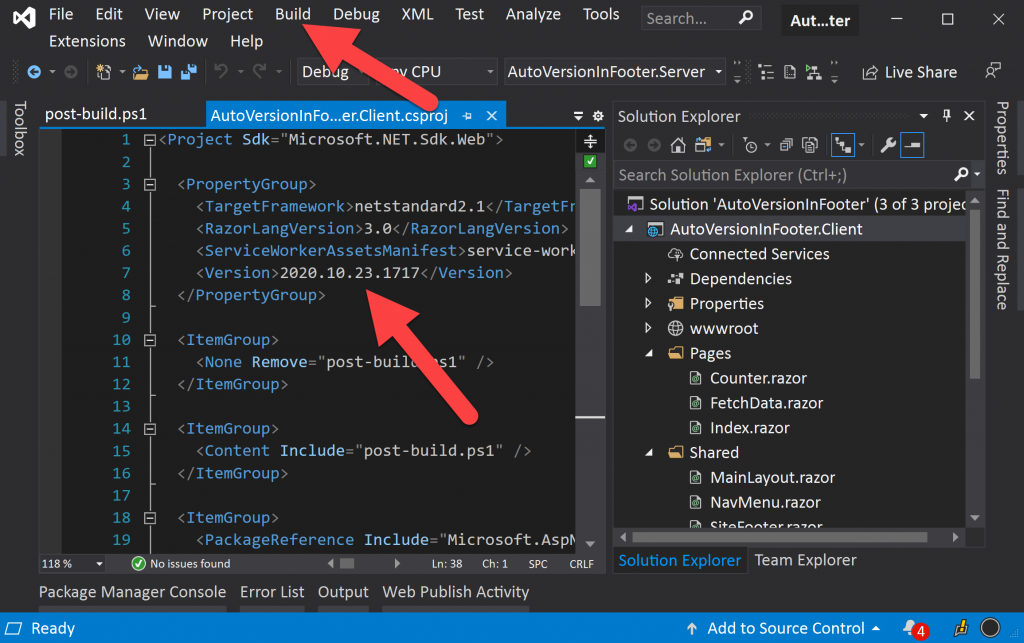
And we have exactly what we want!
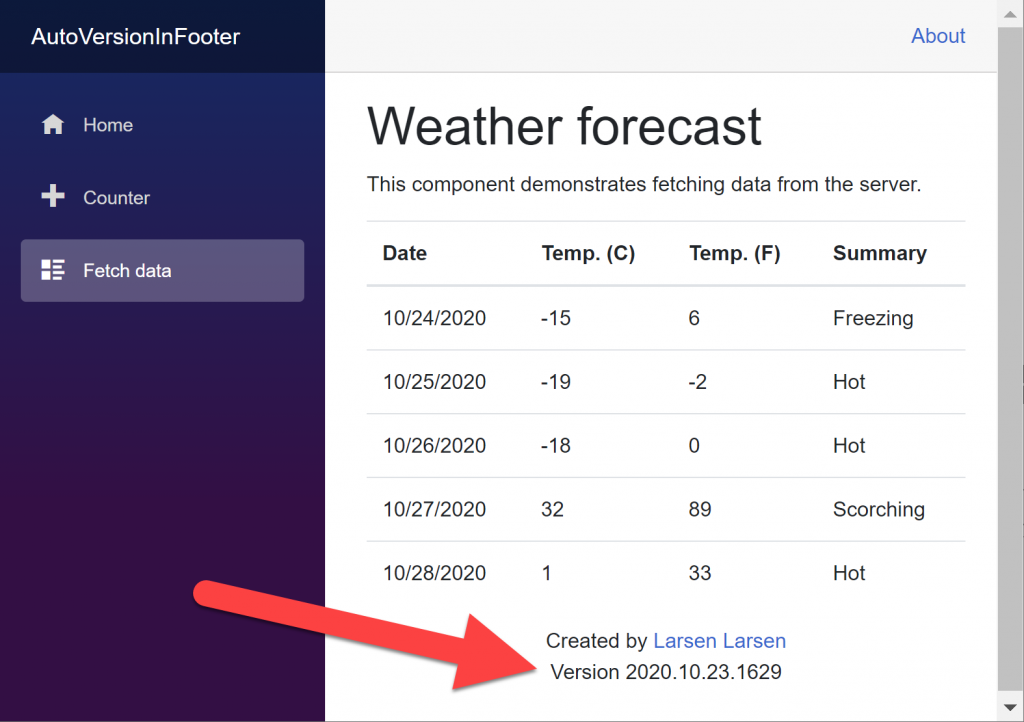
Again, the code sample for this is available here! https://github.com/lancelarsen/AutoVersionInFooter
Good coding! 420 Blazor! :p
Excellent, it works perfectly. Thanks 🙂
In Visual Studio 2022, in the .csproj file
$([System.DateTime]::Now.ToString(yyyy.MM.dd.HHmm))
HH for 24hours, I’m french.
And that’s all.
This is much simpler, and it works perfectly. Thanks!
Perfect, Thx So MUCH …
I followed your tutorial and it works great. Thanks…
But how do I publish the Blazor server project with VS2022 Publish command and not include the post-build.ps1 on the hosting providers server?
Excelent, great solution, thanks a lot sir!